**Assignment 01: Blob to Image (Learning C)** This assignment will introduce you to the C language and to give you practice with data representations in C. In many ways, the C language is like Java. The syntax for variable and function declarations, assignment statements, `for` and `while` loops, and `if`-statements are the same in both languages. The big difference is that in C we have a different view of data, one that is closer to the actual hardware (or at least how you should think of the hardware). We must be aware of where in memory values are located and how much space they occupy. The assignment will give you practice in thinking about variables and pointers, and how they relate to addresses and values in memory. You must work in teams of two (or three if we have an odd number). You may choose your own partner(s). # Learning Goals - Learn how to use Pomona HPC servers - Learn how to use the command-line interface (CLI) - Get started writing, compiling, and running C programs # Grading Walk-Throughs This assignment will be graded as "Nailed It" / "Not Yet" by a TA. To pass ("Nailed It") the assignment, you must 1. Complete the assignment and submit your work to gradescope. + You should start this assignment in class on the day shown on the calendar. + *Complete the assignment as early as possible*. 1. Schedule a time to meet with a TA. You can meet with them after the submission deadline. + You must book a time to meet with a TA + Sign-up on the Google Sheet *with at least 36 hours of notice*. + Contact your TA on Slack after signing up. + All partners must meet with the TA. If you cannot all make it at the same time, then each of you needs to schedule a time to meet with the TAs. 1. Walk the TA through your solutions prior to the deadline. + Walk-throughs should take no more than 20 minutes. + You should be well prepared to walk a TA through your answers. + You may not make any significant corrections during the walk-through. You should plan on making corrections afterward and scheduling a new walk-through time. Mistakes are expected---nobody is perfect. + You must be prepared to explain your answers and justify your assumptions. TAs do not need to lead you to the correct answer during a walk-through---this is best left to a mentor session. 1. The TA will then either + mark your assignment as "Nailed It" on gradescope, or + mark your assignment as "Not Yet" and inform you that you have some corrections to make. 1. If corrections are needed, then you will need to complete them and then schedule a new time to meet with the TA. + You will ideally complete any needed revisions by the end of the day the following Monday. If you have concerns about the grading walk-through, you can meet with me after you have first met with a TA. # Overview For this assignment, you will write code that converts a provided file into an image. You will 1. login to the server (using `ssh` or the Visual Studio Code [Remote - SSH](https://marketplace.visualstudio.com/items?itemName=ms-vscode-remote.remote-ssh) extension), 2. create a new C program that converts a blob of data into a PPM file, and 3. then use [ImageMagick](https://imagemagick.org/) to convert the PPM into a PNG. The following diagram shows the process that I went through and the process that you'll need to complete. I wrote and provided code for `ppm2blob` and you will need to write code for `blob2ppm`. I show in the diagram that a PPM file stores image pixels in an alternating red-green-blue-red-green-blue... format. My `ppm2blob` tool converts this into a red-red-red...green-green-green...-blue-blue-blue format. I also changed the file header. 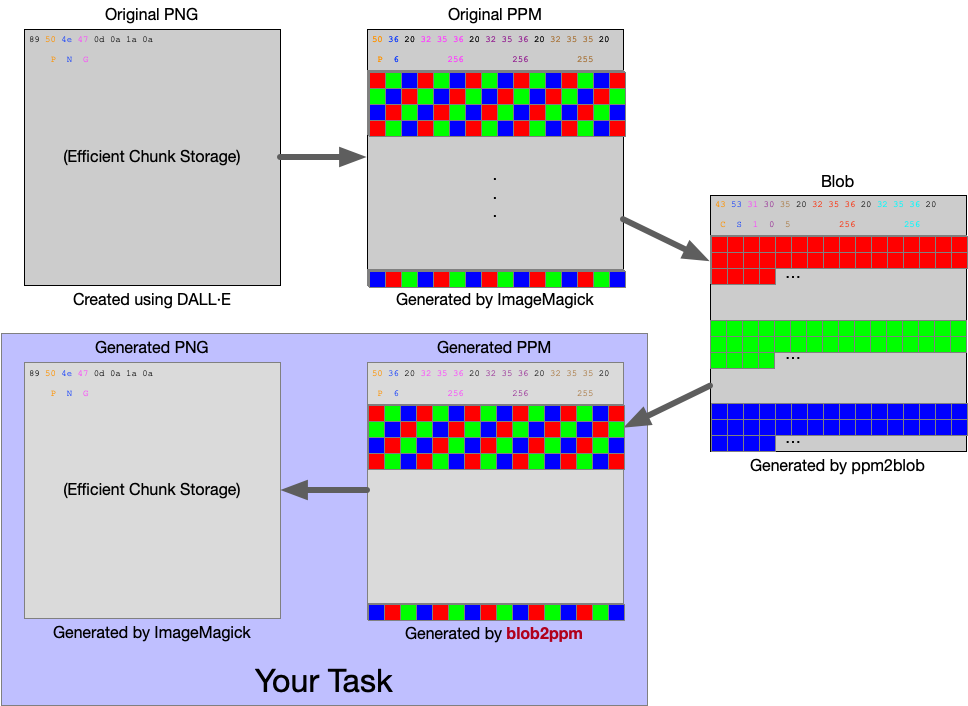 Here is an example showing the behavior of the correct solution. **Do not start coding until you get to the next section.** (You can copy and past from the animation!) If you do not see an animation above this line (or if you see the animation but you don't see the progress bar), you will need to refresh the page (sometimes more than once). Or you can go directly to the player: https://asciinema.org/a/518378 If your program runs correctly, you see a beautiful PNG image that I had [DALLĀ·E](https://labs.openai.com) generate for this assignment using the following phrase. > "A pretty picture for a computer systems programming assignment" # Logging into the server If you are **not** on Pomona's WiFi or Ethernet, you will first need to connect to the Pomona VPN. If you do not already have the Pomona VPN set up on your machine, there are [instructions for how to set-up the VPN here](https://servicedesk.pomona.edu/support/solutions/articles/18000021757). Once you are on Pomona's network, you should `ssh` into the course VM using the following command and your Pomona CAS username and password ~~~bash ssh USERNAME@itbdcv-lnx04p.campus.pomona.edu ~~~ Here is [a nice reference for command line commands](https://cs.pomona.edu/classes/cs105/assignments/commandline.pdf). You can alternatively use Visual Studio Code and the [Remote - SSH](https://marketplace.visualstudio.com/items?itemName=ms-vscode-remote.remote-ssh) extension to access the server. # Accessing the starter files Starter code is available on the server. Use the following commands to make a copy of the starter code. ~~~bash # Create a directory in which to store your assignment mkdir -p ~/cs105/assignments/ # Navigate to that directory cd ~/cs105/assignments # Copy and unpack the starter code tar xvf /data/A01-Blob2Image.tar ~~~ For any command above, you can use [explainshell.com (match command-line arguments to their help text)](https://explainshell.com/) to get a pretty detailed explanation. For example, 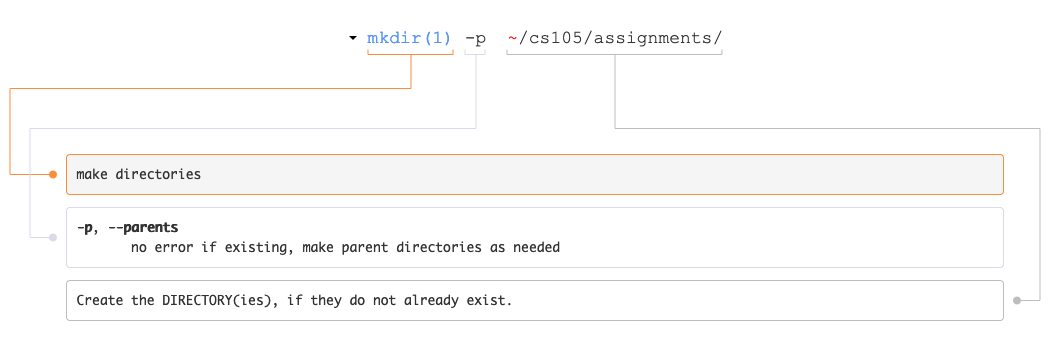 You should now have the following files in a directory named `A01-Blob2Image` - `image.blob`: this is the blob file that I generated from a PPM - `ppm2blob.c`: I created this file to convert the original PPM into a blob (you should reference this file when you get stuck) Let's poke at these files a bit to start. Try the following commands: ~~~bash # Check the file info on the blob file file image.blob # Check the contents of this "data" file (notice anything?) hexyl -n 32 image.blob # Check the file info on the source file file ppm2blob.c # View the contents of the source file (type 'q' to quit the bat program) bat ppm2blob.c ~~~ # Converting the blob to a PPM image Your first task is to write a C program named `blob2ppm.c` that converts my made-up blob file into a valid [PPM](http://netpbm.sourceforge.net/doc/ppm.html) file (a simple, inefficient format for storing an image). Here are the steps involved: 1. Open the `image.blob` file with [fopen](https://en.cppreference.com/w/c/io/fopen). 1. Read the header (all non-pixel data) of the blob file with [fscanf](https://en.cppreference.com/w/c/io/fscanf). 1. Create three arrays (red, green, and blue pixel data) using [malloc](https://en.cppreference.com/w/c/memory/malloc). Here is a guide for [arrays in C](https://www.cs.swarthmore.edu/~newhall/unixhelp/C_arrays.html). 1. Store data from the file into the three arrays with [fread](https://en.cppreference.com/w/c/io/fread). 1. Close the blob file with [fclose](https://en.cppreference.com/w/c/io/fclose). 1. Open/create the `image.ppm` file with [fopen](https://en.cppreference.com/w/c/io/fopen). 1. Write the PPM header with [fprintf](https://en.cppreference.com/w/c/io/fprintf). See the [PPM](http://netpbm.sourceforge.net/doc/ppm.html) and my code for more information on the header. (Hint: the maximum pixel value is `255`). 1. Write the "interleaved" pixel data (r,g,b) with [fputc](https://en.cppreference.com/w/c/io/fputc). 1. Close the PPM file with [fclose](https://en.cppreference.com/w/c/io/fclose). 1. Free the allocated memory with [free](https://en.cppreference.com/w/c/memory/free). Use the provided `ppm2blob.c` file as a guide for each of these steps; the [C Programming Wikibook](https://en.m.wikibooks.org/wiki/C_Programming) is another good resource for general information writing and reading C programs. # Converting the PPM image to a PNG Your next task is to convert the PPM image into a PNG. PNGs are quite a bit more complex and efficient. For this step you'll use an existing tool called [ImageMagick](https://imagemagick.org/). Run the following command: ~~~bash convert image.ppm image.png ~~~ The `convert` utility knows about both PPM and PNG formats. It doesn't know about my made-up blob format. No compare the file sizes of the two files: ~~~bash # Example using ls ls -lh # Example using wc wc -c image.blob wc -c image.ppm wc -c image.png ~~~ # Submitting Your Assignment You will submit your code and/or responses on gradescope. **Only one partner should submit.** The submitter will add the other partner through the gradescope interface.